How to Optimize Performance for ReactJS Applications
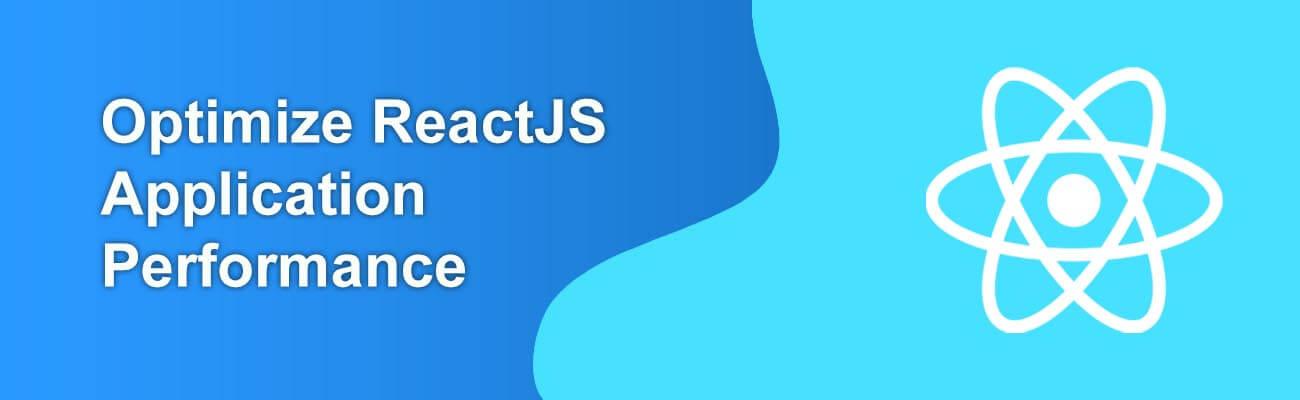
Already have an application developed with ReactJS? If your answer is yes, congratulations! However, it is crucial to improve and deliver continuous lightning-fast user experiences that will keep your users coming back. Fortunately, there are several ways to improve the performance of your already-worthy ReactJS application.
In this article, we will dive deep into effective performance optimization techniques that will elevate your application to the next level. So, let’s get ready to take your ReactJS app to new heights of performance and enhance your user experience.
10 Effective Performance Optimization Techniques in ReactJS
Let’s take a quick dive into the 10 most effective techniques that take your application to the next level and provide your users with a smooth and efficient user experience.
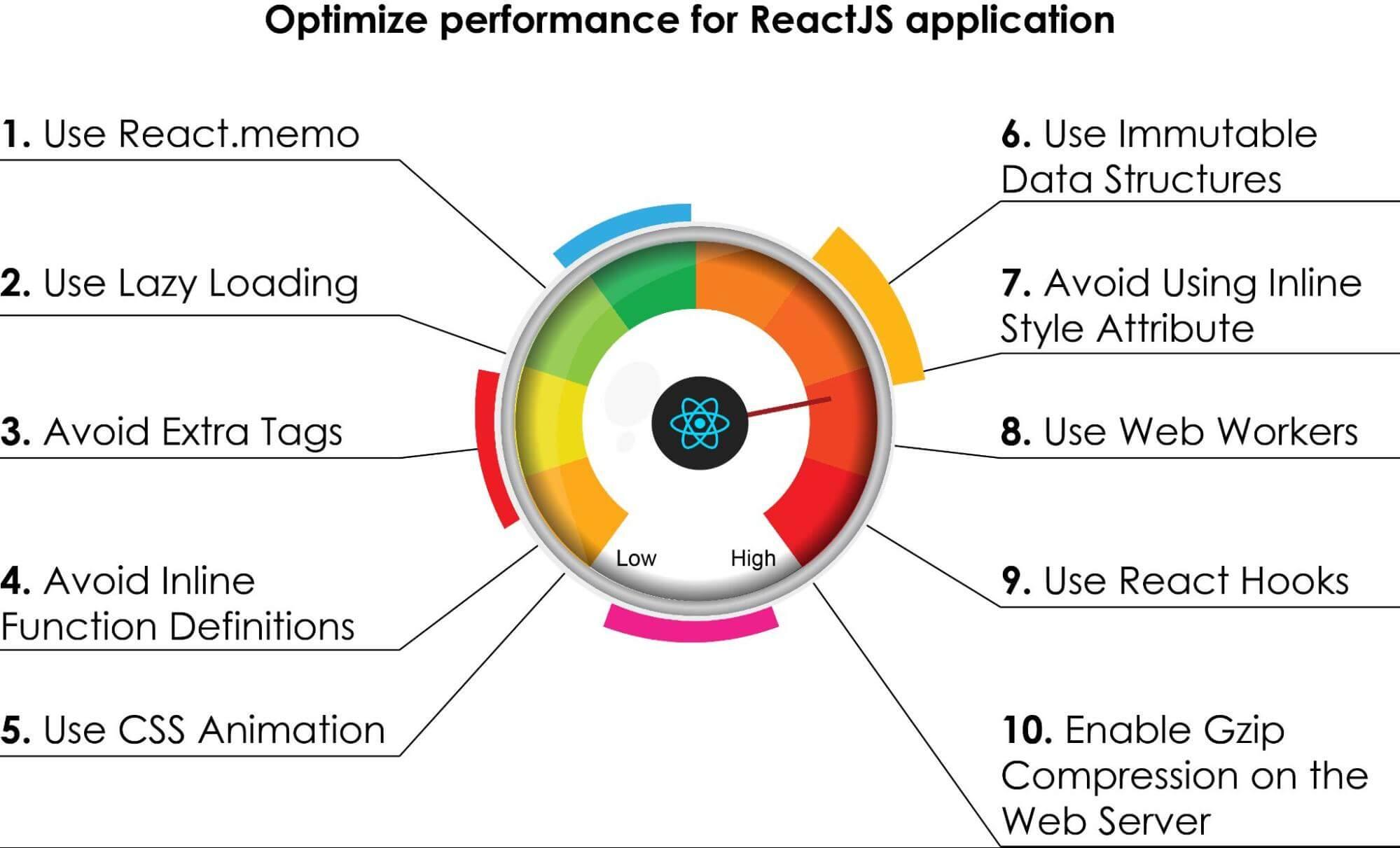
Using React.memo
React.memo is used for component memoization. It skips re-rendering the component if the state is the same. For example, if a user selects the same item repeatedly from a list, you can skip rendering the component based on that list item. This improves application performance. Below, we share an example of React.memo
function RenderingComponent(props) { return ( <div> <b>User name: {props.name}</b> <b>User id: {props.id}</b> <b>User status: {props.status}</b> </div> ) } var memoComponent = React.memo(RenderingComponent);
Let’s break the code and see how it works. In the example above we first created a function named RenderingComponent(props) with parameter props. Now props is an object reference passed to the function that contains the name, id, and status of a particular user.
After that we created a variable named memoComponent with React.memo and passed our function RenderingComponent as a parameter. Now when any user provides the same name, id, and status to the application, the React.memo will compare the previous value and current value of name, id, and status. If they are the same then it will skip rendering the UI.
In this way, your component will not re-render if the user provides the same information to the system again and again.
Using Lazy Loading
If your application includes large files like images or videos, it can cause long rendering times and delay the user’s experience. By splitting the required files based on their need, you can reduce loading time and load components only when necessary. This process, known as lazy loading, creates a faster and more efficient application. In ReactJS, you can use Suspense and lazy for this purpose. react-lazyload and react-lazy-load-image-component are the two mostly used Lazy Loading libraries for ReactJS.
import { LazyLoadImage } from "react-lazy-load-image-component"; export default function App() { return ( <div> <LazyLoadImage src={"https://example.com/dir/toimage"} width={700} height={600} alt="Alt for the image" /> </div> ); }
In our above example, we first import the LazyLoadImage from the react-lazy-load-image-component library. After that, we just need to pass our image details like location, width, and height to the <LazyLoadImage /> tag. That’s it, we are done!!!
Avoiding Extra Tags
Extra tags are necessary when multiple elements are present in a render() function. These tags create a complex code structure and make application updates more difficult. To resolve this, use React fragments instead of extra tags. Fragments provide a parent for adjacent tags without creating additional elements, making the code more maintainable.
Here is an example showing how you can use React Fragment,
class Sample extends React.PureComponent{ render() { return ( <React.Fragment> <h1>My Heading</h1> <p>My comments</p> </React.Fragment> ); } }
You can also do the same thing as below,
class Sample extends React.PureComponent{ render() { return ( <> <h1>My Heading</h1> <p>My comments</p> </> ); } }
The only difference is we just replaced the <React.Fragment></React.Fragment> with the <></>.
Avoid Inline Function Definitions
Using inline functions in the render() function creates new function instances with each render, impacting application performance. Instead, avoid inline function definitions to prevent unnecessary re-binding and improve performance.
Here is an example containing an inline function definition,
import React from "react"; export default class InlineFunctionDef extends React.Component { render() { return ( <div> <input type="button" onClick={(e) => { this.setState({inputValue: e.target.value}) }} value="Click Here" /> </div> ) } }
In the example above we created an inline function for our button. As we already discussed this will make your application slower. To avoid inline function definition, we just need to create the function separately and pass it to the place where it’s necessary. Have a look at the optimized version of our above example,
import React from "react"; export default class InlineFunctionDef extends React.Component { setNewData = (event) => { this.setState({ inputValue: e.target.value }) } render() { return ( <div> <input type="button" onClick={this.setNewData} value="Click Here" /> </div> ) } }
Use CSS Animation
JavaScript-based animations require continuous CPU processing, which can increase CPU usage and cause a sluggish user experience. Additionally, complex animations with many components can further degrade performance and increase code complexity. To address this, favor CSS animations over JavaScript animations for improved overall application performance.
Using Immutable Data Structures
Immutable data structures involve creating a copy of an object, comparing and identifying changes, and updating only when necessary. When users repeatedly select the same items, immutable data structures prevent unnecessary reloading of the same object. This approach ensures a smooth user experience by loading and updating the object only when required. Take a look to the below example,
const [UserInfo, setUserInfo] = useState({ name: "Olduser name", noOfPages: 30 }); const updateUserInfo = () => { const newUserInfo = { ...UserInfo }; newUserInfo.name = "NewUser name"; setUserInfo(newUserInfo); };
Let’s explain the above code. We first created a variable named newUserInfo that copy the current state of UserInfo. After that we updated the username and passed it to setUserInfo. In this way the application can track the change in UserInfo and update the UI when necessary.
Avoid Using Inline Style Attribute
Inline style attributes in HTML elements require additional scripting and rendering time, impacting application loading time. Maintenance of CSS styling also becomes more challenging. Utilizing an external CSS file for defining styles is preferable, as it improves application performance and simplifies maintenance.
Use Web Workers
Web workers are beneficial for executing CPU-intensive tasks in web applications. JavaScript, being single-threaded, handles synchronous executions, leading to delays in rendering tasks. By employing web workers, CPU-intensive tasks are executed in isolated environments, allowing the main thread to focus on other tasks. This results in a more efficient and faster application.
Use React Hooks
React Hooks allow for the utilization of state and other React features in functional components, enhancing code readability, maintainability, and reusability. Hooks simplify complex logic, reduce cognitive load, and facilitate easier component writing and testing.
Enable Gzip Compression on the Web Server
Gzip compression reduces the size of large files, such as images, videos, and texts, during network transfers. Enabling gzip compression on the web server saves network bandwidth and accelerates application loading by minimizing data transfer sizes.
Upgrade your application to the next level
ReactJS allows for faster development, improved user experiences, and increased customer satisfaction. The reusable code and robust ecosystem enable scalability and cost-effective maintenance. In this comprehensive article, we explored some effective techniques to improve your ReactJS application. By utilizing these techniques, you can elevate your application to a higher level of performance, providing your users with a 5-star experience.
These techniques also address issues such as loading time, slow rendering, laggy UI, and other performance-related concerns. If you’re wondering how to select the best techniques for your application, don’t worry. We are here to assist you in identifying and implementing the optimal strategies tailored to your ReactJS application. Contact us when you’re ready to discuss further enhancements.