A Native PHP Function Override with namespace & PHP-Mock: Shopify API Example
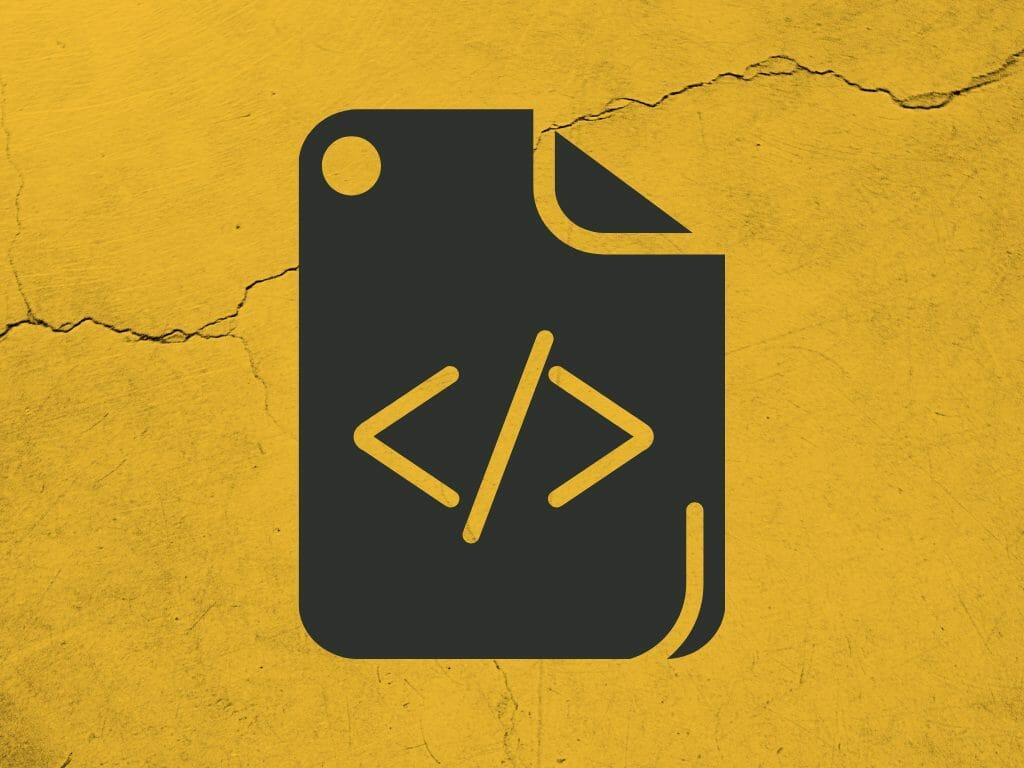
The Problem and the PHP Function Relationship
The PHPShopify library is great for common Shopify API integration needs in customizing your project. However, it doesn’t provide the direct Shopify API response to your code and lacks extension points to retrieve that raw API response. Let’s see why a PHP override function is necessary to overcoming the problem.
In our application, we needed to get at the raw Shopify API response data in order to create detailed debug logs, for instance, when we hit Shopify’s API rate limit. In this article, we’ll show you how to override native PHP functions using a trick made possible by PHP’s namespacing feature and PHP-Mock.
What is PHP-Mock?
PHP-Mock is a library that is primarily used in a testing environment to override the definition of a native PHP function that is called in a namespaced file. A small example given by the library shows a basic idea of when this library can be used: