Wordpress Custom Post Types
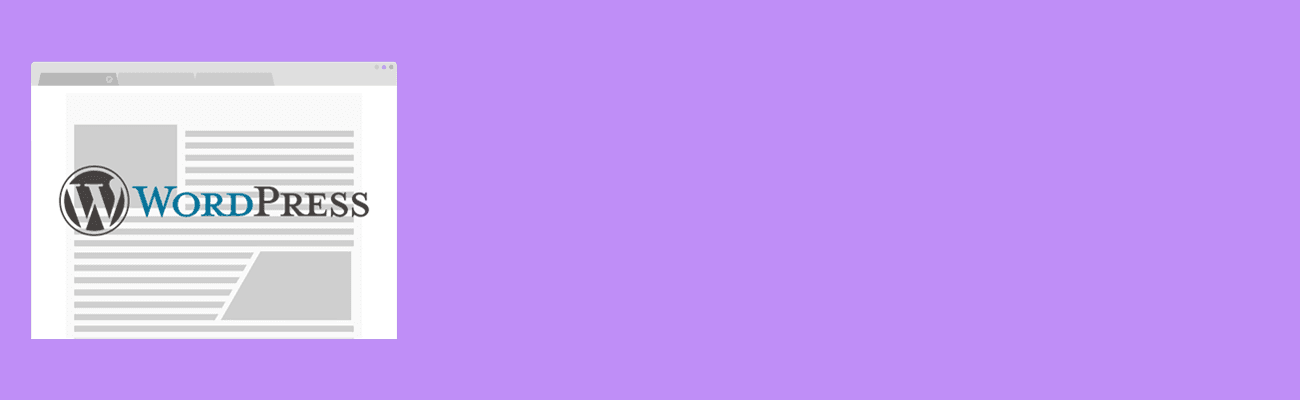
What is a Custom Post Type?
WordPress is an open source content management system that allows developers to create a wide array of plug-ins, themes and widgets. One of the core pieces in WordPress is creating Custom Post Types. A Custom Post Type is an easy way to organize and create data that is stored in the database.
Why is it useful?
Creating a custom post type is useful visually and structurally because it creates a common place where you can distinguish two very different types of content. Say you want an easier way to organize posts and portfolio pieces; by adding a custom post type of ‘portfolio,’ you can then store all portfolio pieces in a location separate from blog posts.
Creating a Custom Post Type
Custom Post types are created in two ways: either in the functions.php file or in a plugin. This example is created in a plugin, which I believe is most useful, as it can be used in multiple themes.
To create a post type you will have to use the register_post_type function which allows you to add arguments to your post type. Below is the code to create a custom post type of Portfolio items.
function ep_register_post_type() { // Create Portfolio Custom Post Type //$labels - These labels are for the Backend of your WordPress site it overwrites the word 'post' $labels = array( 'name' => 'Portfolio', 'singular_name' => 'Portfolio', 'menu_name' => 'Portfolio', 'name_admin_bar' => 'Portfolio', 'add_new' => 'Add New', 'add_new_item' => 'Add New Portfolio', 'new_item' => 'New Portfolio', 'edit_item' => 'Edit Portfolio', 'view_item' => 'View Portfolio', 'all_items' => 'All Portfolio', 'search_items' => 'Search Portfolio', 'parent_item_colon' => 'Parent Portfolio:', 'not_found' => 'No portfolio found.', 'not_found_in_trash' => 'No portfolio found in Trash.', ); $args = array( 'labels' => $labels, 'public' => true, // this needs to be set to true and controls bottom 3 'publicly_queryable' => true, //queries can be performed on the front end for the post type 'show_ui' => true, //generate and allow a UI for managing post type 'show_in_menu' => true, //show the post type in the admin menu 'menu_icon' => 'dashicons-screenoptions', //supports these dashicons to be displayed on menu //dashicons url: https://developer.wordpress.org/resource/dashicons/#editor-insertmore 'query_var' => true, //quieries can be performed 'capability_type' => 'post', //build the read, edit, and delete capabilities 'has_archive' => true, //there should be post type archives 'hierarchical' => false, //whether the post type is hierarchical 'menu_position' => 5, //position in the menu order 'rewrite' => array( // *** Important *** Triggers the handling of rewrites for this post type. 'slug' => 'portfolio', // customize the slug ex. endertech.com/portfolio/*custom-post* 'with_front' => false //add for ^ example url ), 'taxonomies' => array('category', 'post_tag'), // Wordpess support of categories and tags 'supports' => array('title', 'editor', 'thumbnail', 'custom-fields', 'excerpt', 'revisions') //Wordpress supports ); register_post_type('ep_portfolio', $args); /*** Important url: https://developer.wordpress.org/reference/functions/register_post_type/ allows for a custom post type to be created ***/ } add_action('init', 'ep_register_post_type'); //Flushing rewrite automatically tells wordpress to use the slugs uptop /** Important **/ //Do not forget to update the permalinks in the backend function my_rewrite_flush() { ep_register_post_type(); flush_rewrite_rules(); } register_activation_hook(__FILE__, 'my_rewrite_flush');
Displaying Custom Post Type – Archive/Single Pages
Now that you have created the custom post type, ‘ep_portfolio,’ for Portfolio items, you might wonder how can we display the portfolio items? In the WP Hierarchy, it explains by creating a custom post type we have to create an
archive-$posttype.php and a single-$posttype.php. This is where we will have our loop pull in our custom post types. In this case, I will have to create a archive-ep_portfolio.php page for the portfolio archive page and a single-ep_portfolio.php page for the portfolio detail page.
Querying Custom Post Types
WP_Query function allows you to query the database and retrieve your custom post type. There are multiple arguments you can add to make your query as detailed as possible. There is a list of the arguments at codex.
A basic example of WP_Query that displays all portfolio items that are published in ascending order.
<?php // define query parameters $args = array( 'post_type' => 'ep_portfolio', //your custom post type 'post_status' => 'publish', //only published posts 'order' => 'ASC', //in ascending order 'posts_per_page' => -1, //select all posts ); // run the loop based on the query $portfolio_posts = new WP_Query($args); if ($portfolio_posts->have_posts()) { while ($portfolio_posts->have_posts()) { $portfolio_posts->the_post(); ?> <!-- displays the title and the content --> <h2><?php the_title(); ?></h2> <div><?php the_content(); ?></div> <?php } wp_reset_postdata(); } else { echo '<p> Posts Not Found </p>'; } ?>
Endertech is a Los Angeles WordPress Developer company able to provide solutions for your WordPress and other web development needs. Contact us for your free consultation.