Hydrogen Components, Hooks, and Utilities Explained
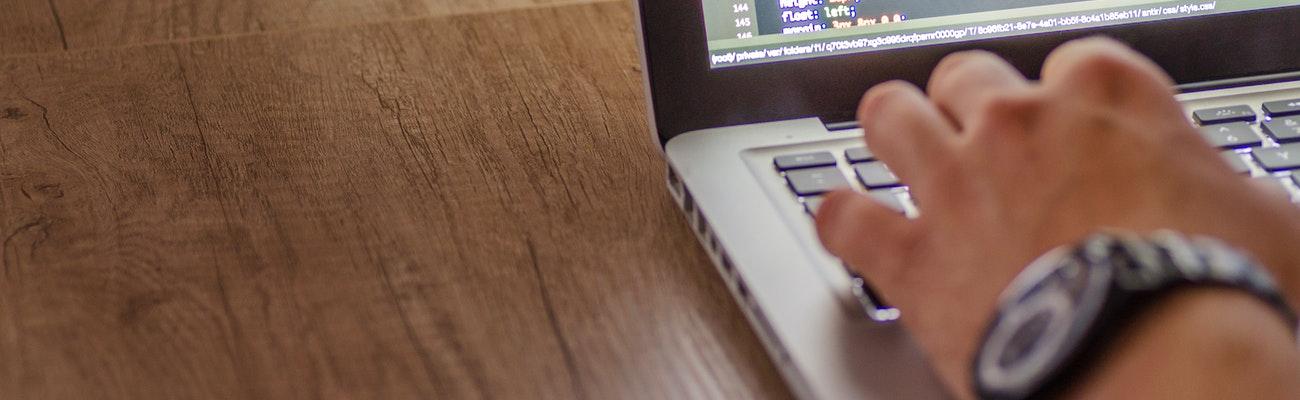
Until recently, Shopify developers designing headless storefronts were forced to build custom components for tasks such as pulling product data or adding products to the cart.
To overcome this challenge, Shopify launched Hydrogen, an opinionated frontend design framework based on React.
Previously, we described how Shopify uses the Hydrogen framework and React Server Components to accelerate frontend design. Here, we’ll explore how Hydrogen’s components, hooks, and utilities integrate its React-based frontend with Shopify’s backend.
React-Specific Concepts You Should Know
Familiarizing yourself with the following concepts will help you understand how hooks, components, and utilities work in Shopify Hydrogen as you read the rest of our guide.
Function: Function is a block of code that performs a specific task. Functions make it easier to reuse existing code blocks to perform different tasks in React. Class: Class is a special type of function used to create collections of properties consisting of key-value pairs. Classes are used in object-oriented programming languages such as JavaScript to simplify development using modular code blocks. State: State is code inside a React component that defines the component’s behavior and how it renders. It makes React components dynamic and interactive. A React component with state is also called a stateful component. Props: Props, or properties, are read-only components that exist outside a React component and pass arguments into React components. Routes: Routes are components displayed conditionally based on user interactions.
Hydrogen Components
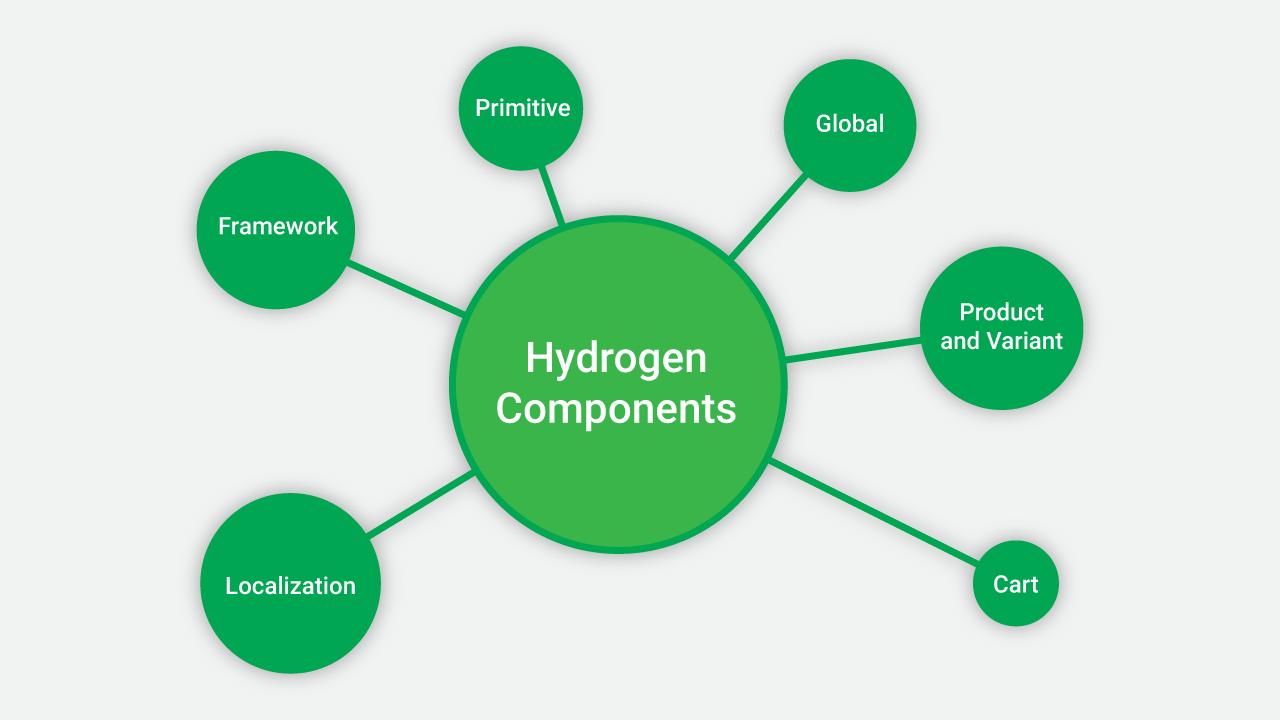
Hydrogen components are objects containing the business logic for different commerce functionalities. In other words, they’re functions that divide the storefront into isolated yet reusable pieces of code that can parse and process data.
Shopify broadly categorizes Hydrogen components into six role-based categories as follows:
Framework
Primitive
Global
Product and variant
Cart
Localization
Here’s a quick overview of each Hydrogen component type.
Framework Components
Framework components are pieces of code that perform tasks related to the framework, such as building and navigating routes. Hydrogen includes the following four framework components:
FileRoutes
Link
Route
Router
Primitive Components
Primitive components are the building blocks for various frontend component types, including products, variants, and cart. They perform storefront-related tasks such as rendering SEO information, media, and the Shop Pay checkout button.
Hydrogen includes the following ten primitive component types:
ExternalVideo
Image
MediaFile
Metafield
ModelViewer
Money
Seo
ShopPayButton
UnitPrice
Video
Global Components
Global components are code blocks that relate to the entire app. Hydrogen includes one global component called ShopifyProvider that spans the whole app and allows you to use hooks.
ShopifyProvider only works with the Hydrogen frameworks and cannot be used with other frameworks such as Next.js.
Product And Variant Components
Product and variant components render code related to a product and its variants, such as descriptions, pricing, titles, and metafields.
Hydrogen includes the following five product and variant components:
ProductDescription
ProductMetafield
ProductPrice
ProductProvider
ProductTitle
Cart Components
Cart components are code blocks that render the elements on the cart page, such as the cart line price, product information, and the Shop Pay button.
Hydrogen includes the following 13 cart components:
AddToCartButton
BuyNowButton
CartCheckoutButton
CartEstimatedCost
CartLineImage
CartLinePrice
CartLineProductTitle
CartLineProvider
CartLineQuantity
CartLineQuantityAdjustButton
CartLines
CartProvider
CartShopPayButton
Localization Components
Localization components are pieces of code that render location-specific content. Hydrogen currently includes one localization component called LocalizationProvider.
This component queries the Storefront API and stores the information about the user’s isoCode and country name to localize frontend content.
Hydrogen Hooks
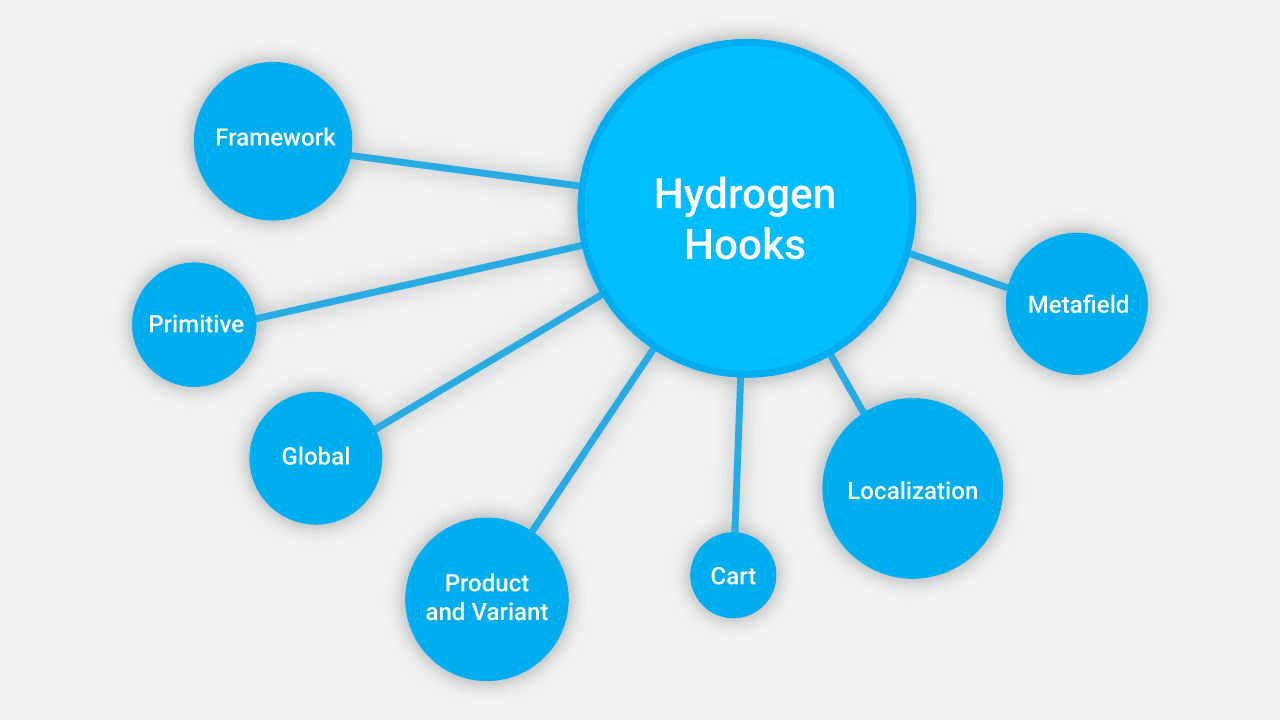
Hydrogen hooks are built-in functions in Hydrogen that let you “hook into” or access the state inside a Hydrogen component. Thus, you can reuse business logic stored inside a component without altering the entire component hierarchy.
There are seven Hydrogen hook categories as follows:
Framework
Primitive
Global
Product and variant
Cart
Localization
Metafield
Here’s a quick overview of the different Hydrogen hooks.
Framework Hooks
Framework hooks in Hydrogen navigate routes and fetch parameters from an active route.
Shopify provides two Hydrogen hooks — useNavigate and useRouteParams. useNavigate is only available in client components, whereas useRouteParams is available in both client and server components.
Primitive Hooks
Primitive hooks are fundamental units of different component types such as product, variant, and cart. There are two primitive hooks in Hydrogen — useLoadScript and useMoney.
useLoadScript loads external script tags on the client-side. It returns values such as loading, done, and error to help you understand the status of the external script. The useMoney hook returns a pre-formatted string of a monetary value and currency indicator.
Global Hooks
Global hooks relate to the entire Hydrogen app and can fetch data from server-side components. Hydrogen provides the following six global hooks:
fetchSync
useQuery
useServerState
useShop
useShopQuery
useUrl
Product and Variant Hooks
Hydrogen includes two product and variant hooks — useProduct and useProductOptions. These hooks relate to products (and their variants) and help populate product information on the storefront.
The useProduct hook returns the product title, description, media, variants, and price information. The useProductOptions returns data specific to a variant, such as stock and selling plan information.
Cart Hooks
Hydrogen includes two cart hooks — useCart and useCartLine — to provide access to cart and cart line objects.
The useCart hook allows access to the data, such as the cart’s checkout URL and discount codes applied. The useCartLine hook returns information about cart line items such as their ID, quantity, and attributes.
Localization Hooks
Localization hooks return a finite ordered list of data containing the current country and function to update it. Hydrogen uses a single localization hook called useCountry. It returns two elements — the country’s isoCode and name at index value 0, and a function to update the country at index value 1.
Metafield Hooks
Metafield hooks return an array of metafields whose values have been parsed according to the metafile type. Hydrogen includes a metafield hook called useParsedMetafields to perform this action.
Hydrogen Utilities
Utilities are general-purpose functions used to improve the development process. Hydrogen includes the following six utilities to accelerate app development:
flattenConnection
log
isClient
isServer
parseMetafieldValue
queryShop
The above utilities can be used to debug Hydrogen apps, identify whether a piece of code was run on the client or server, and query the Storefront API.
Hydrogen Components, Hooks, and Utilities: How It All Comes Together
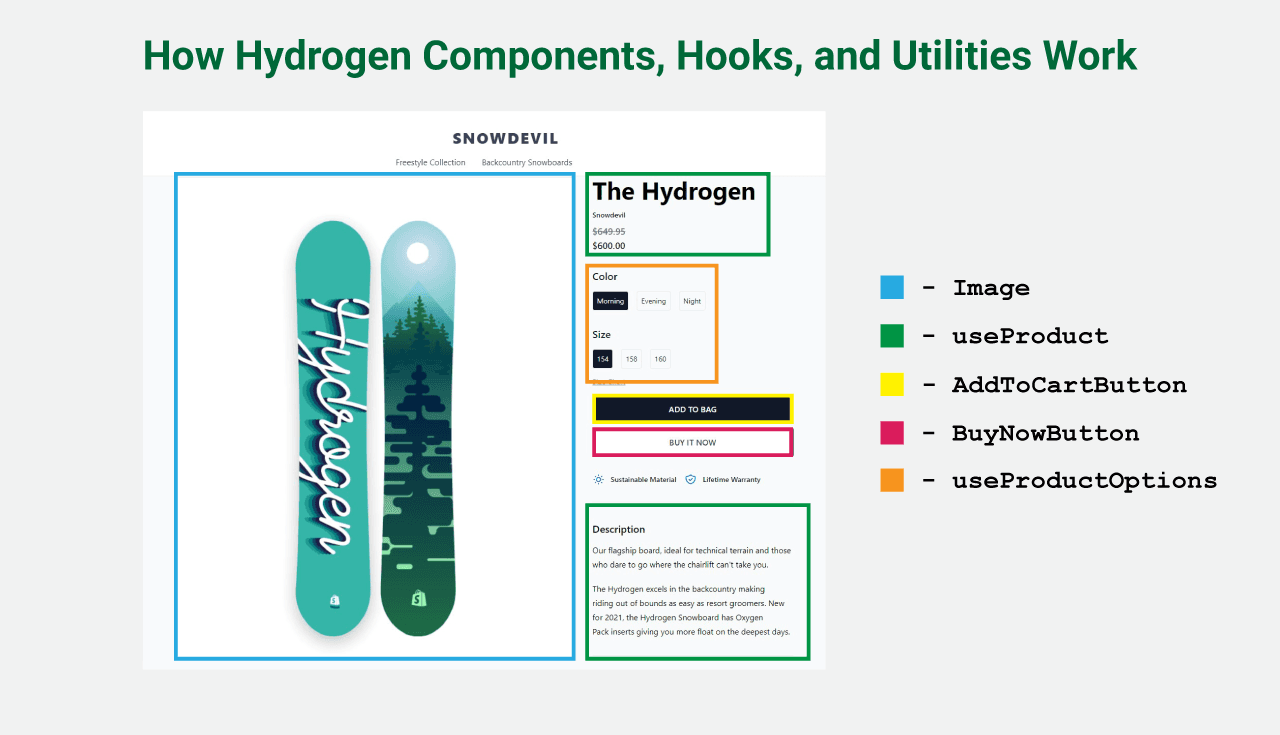
The primary goal of providing components, hooks, and utilities in Hydrogen is to facilitate faster development of custom storefronts. Here’s a high-level view of how it all works together:
If you want to build a product page in Hydrogen, you can start by importing the Layout component from Shopify’s demo template.
Then, using the client-side ProductProvider component, you can set up a context (information sharing between components) with product details. With the context in place, add product data such as description and price by importing the useProduct hook.
You can also use the useProduct hook and useProductOptions component to create a variant dropdown. At the same time, for faster computation and better performance, flatten the array of nodes from the Storefront API using the flattenConnection utility.
Finally, you can include an Add to Cart button using the AddToCartButton component to complete the page.
Thus, using a mix of Hydrogen components, hooks, and utilities, you can assemble a fully functional product page for your storefront without starting from scratch.
Shopify Hydrogen: Skip the Mundane, Build Rapidly
Hydrogen’s Shopify-friendly components, hooks, and utilities accelerate the development of dynamic storefronts and eliminate the need for custom GraphQL queries to access Shopify’s APIs. They simplify building customer storefronts with Shopify and allow developers to focus on designing intuitive shopping experiences.
Are you thinking about building a custom storefront with Shopify Hydrogen? Contact our Shopify experts and discover how Hydrogen can help your business grow.