How to easily add a Medium style Image Zoom to your Website, Store, or Blog
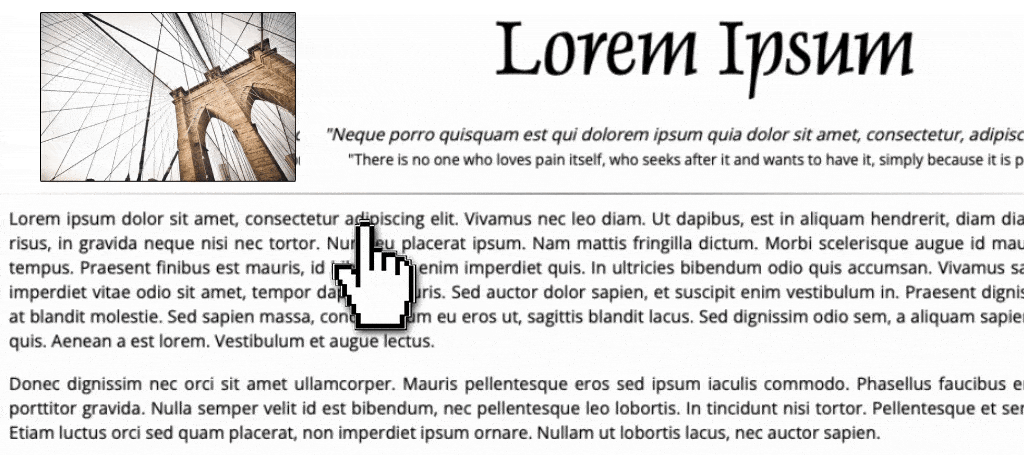
How to easily add a Medium style Image Zoom to your Website, Store, or Blog
The Problem
Recently I was working on a project that had an existing image zoom functionality built in, but for whatever reason it became unreliable in Safari, causing images to appear blurry when zooming. As it turns out, for whatever reason between how this function worked, and how Safari works, it was only expanding a tiny optimized thumbnail to the expected resolution rather than just showing the original full size image.
The Solution
In comes Zoom-Vanilla, an incredibly simple to use library using vanilla javascript to get the job done. The pre-existing solution required us setting up functions to find and attach a zoom instance to each image, what a headache! With Zoom-Vanilla we just need to include some links to the lightweight css and javascript and add a little extra bit of information to our image tags.
https://github.com/spinningarrow/zoom-vanilla.js
Implementation
Below I'm going to provide both the easier and medium (ha!) to hard difficulty implementations.
Easy
The simplest way to get zoom-vanilla working is to follow these steps:
1. Add <link href="https://cdn.jsdelivr.net/npm/zoom-vanilla.js/dist/zoom.css" rel="stylesheet">
between the <head></head>
tags of your page
2. Add <script src="https://cdn.jsdelivr.net/npm/zoom-vanilla.js/dist/zoom-vanilla.min.js"></script>
just before the closing </body>
tag in your page
3. Inside the <img>
tag for your image, add data-action="zoom"
as in: <img src="path-to-image.jpg" data-action="zoom">
(These links are css and javascript files hosted on a CDN or Content Delivery Network. This means that they are more likely to load quickly in more locations)
The final result might look something like this:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>My Awesome Website</title>
<link rel="stylesheet" href="./style.css">
<!-- Add zoom-vanilla.js CSS witin the head tag -->
<link href="https://cdn.jsdelivr.net/npm/zoom-vanilla.js/dist/zoom.css" rel="stylesheet">
</head>
<body>
<main>
<h1>Welcome to My Awesome Website</h1>
<!-- Add data-action="zoom" to the image -->
<img src="path-to-image.jpg" data-action="zoom" alt="Zoomable Image">
</main>
<!-- Add zoom-vanilla.js JavaScript just before the </body> tag -->
<script src="https://cdn.jsdelivr.net/npm/zoom-vanilla.js/dist/zoom-vanilla.min.js"></script>
</body>
</html>
Voila! Open the page and click on the image. You should see it zoom to fill the available window space! Click again, and it should go back to the thumbnail size.
https://codepen.io/IB21-A/pen/KKLWWVB
Medium / Hard
Download the files from the CDN links and link them with the appropriate tags.
Depending on your level of comfort this could be a bit more difficult, but it can also be more reliable as it is not dependent on an external server. Assuming your website has some sort of file structure such as the following:
src/
├── assets/
│ ├── css/
│ │ └── zoom.css
│ └── js/
│ └── zoom-vanilla.min.js
└── index.html
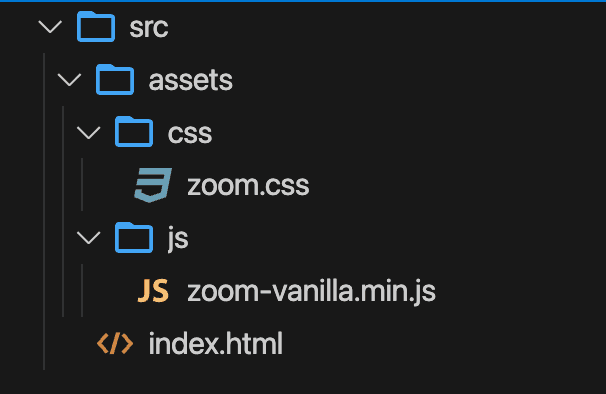
you will want to save the files into the respective folders.
zoom.css
gets saved insrc/assets/css
zoom-vanilla.min.js
gets saved insrc/assets/js
Then just like before, you will want to include the local versions of these files:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Click the image to zoom</title>
<link rel="stylesheet" href="./zoom.css" />
</head>
<body>
<main>
<h1>Click an image to zoom</h1>
<!-- Add data-action="zoom" to the image -->
<img
class="thumbnail"
src="https://picsum.photos/800"
data-action="zoom"
alt="Zoomable Image"
/>
<!-- Add zoom-vanilla.js CSS witin the head tag -->
<link href="./assets/css/zoom.css" rel="stylesheet" />
<!-- Add zoom-vanilla.js JavaScript just before the /body tag -->
<script src="./assets/js/zoom-vanilla.min.js"></script>
</body>
If this is a React app, you may need to place the js file in your public directory and slightly alter the path to use PUBLIC_URL
in order for the compiler to properly locate the file. Adding this script tag to your public/index.html,
just before the closing </body> tag:
<script src="%PUBLIC_URL%/js/zoom-vanilla.min.js"></script>
Will get compiler to find the file at "my-react-app/public/js/zoom-vanilla.min.js"
Likewise you can store the vanilla-zoom.css
file wherever your other css files are and include it in your app.css
using @import url('vanilla-zoom.css'); at the top of your app.css file.