Editable DataGrid with jQuery
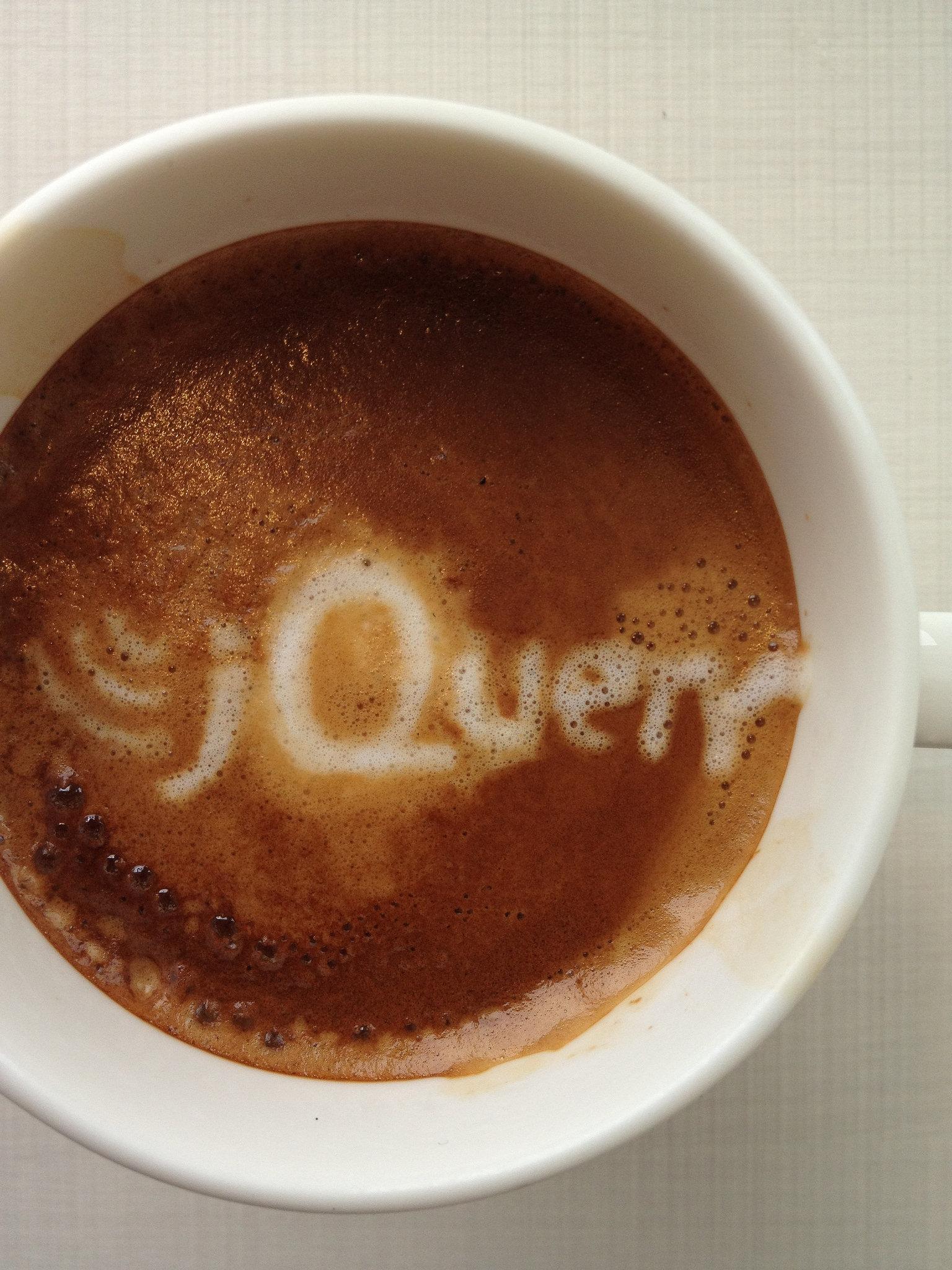
While working with SlickGrid, I wondered how complex would it be to make an editable datagrid from scratch. I surmised that with jQuery this might be a fairly simple task. I was correct.
I started with my html, a simple table where the id uniquely identified each cell within. Here is a snippet of how a row appears:
John
Doe
432 South A. St.
Los Angeles
CA
Note that the id represents the record in the database. In this case 42 is the record id and the number following the period is the column. In the script that processes the edit, the column numbers are mapped to actual field names.
I then came up with the following javascript. I’ll set forth the entire script here and comments within it describe what’s happening and why.
jqTableEditor = {
// A flag that prevents other cells from becoming editable while another cell
// is currently being edited.
txtCellEditorActive: false,
// This sets up listeners on the target datagrid table. Call from '$(document).ready'.
init: function() {
// Capture doubleclick event on a table cell.
$('#tblMain td').live('dblclick', function(event){
// Check flag to see if another td is being edited. if so, abort.
if(jqTableEditor.txtCellEditorActive) {
return;
}
// Get jQuery object for the double-clicked td
var jqo = $(this);
// Add a class that removes the padding
jqo.addClass('tdCellEditor');
// Get the td's width
var width = jqo.width() + 5;
// Create the cell editor's html.
// - set its class so it can be formatted via css
// - set its width to be equal to the td�s width.
// - set value to be that of the td's content
var cellEditor = '<input type="text" value="'+this.innerHTML+'" style="width:'+width+'px" />';
// Set the td�s html to the cellEditor input control
jqo.html(cellEditor);
// Set the flag to indicate an edit is active
jqTableEditor.txtCellEditorActive = true;
// Set the focus to the input control so the user can immediately start editing
$('.txtCellEditor').focus();
});
// Capture ENTER key press in the cell editor <input> control; results in committing the edit.
$(document).on("keyup", '.txtCellEditor', function(e){
var k = (e) ? e.which : event.keyCode;
if(k == 13) {
jqTableEditor.txtCellEditorCommit();
}
});
// Capture BLUR of cell editor <input> control; results in committing the edit.
$(document).on("blur", ".txtCellEditor", function(e){
jqTableEditor.txtCellEditorCommit();
});
},
txtCellEditorCommit: function() {
var tce = $(".txtCellEditor"); // Get the jQuery object for the cell editor <input> control.
var td = tce.parent('td'); // Get the TD being edited.
var value = tce.val(); // Get the value in the cell editor <input>
// Remove cell editor and load the TD with edited value. Also remove the tdCellEditor class from the TD.
td.html(value).removeClass('tdCellEditor');
// save changes via ajax
var id = td.attr('id'); // get the TD�s id
$.ajax({
type: "POST",
url: 'process_cell_edit.php', // script that updates database with change based on TD�s id
data: {id: id, value: value},
success: function(data, textStatus, jqXHR) {
// You can indicate to the user that their edit was saved by flashing a �saved� indicator on the screen.
// The #imgAjaxSaveSuccess is the id of an absolutely positioned image you want to flash (I used a green checkmark).
// Using the offset, the indicator flashes over the edited TD.
var tceOffset = tce.offset();
$('#imgAjaxSaveSuccess').css('top', tceOffset.top).css('left', tceOffset.left).show().fadeOut(750);
},
error: function(jqXHR, textStatus, errorThrown) {
alert('error: '+textStatus);
}
});
jqTableEditor.txtCellEditorActive = false; // Finally, clear the flag that an edit is taking place
}
}
// once the document has loaded, initialize the table and set event listeners on the tds.
$(document).ready(function() {
jqTableEditor.init();
});
That’s it! A fully functional datagrid editor in ~100 lines of code thanks to jQuery. Here’s the accompanying CSS:
#tblMain {
margin-top:10px;
border-collapse: collapse;
}
#tblMain th,
#tblMain td {
border:1px solid #333;
font-size:11px;
font-family:verdana;
height:24px;
padding:0 3px;
}
/* CELL EDITOR */
.tdCellEditor,
#tblMain td.tdCellEditor {
padding:0 1px;
}
.txtCellEditor {
font-size:11px;
font-family:verdana;
}
EnderTech is a Los Angeles Web Developer able to provide solutions for your Software Development needs. Contact us for a free consultation.
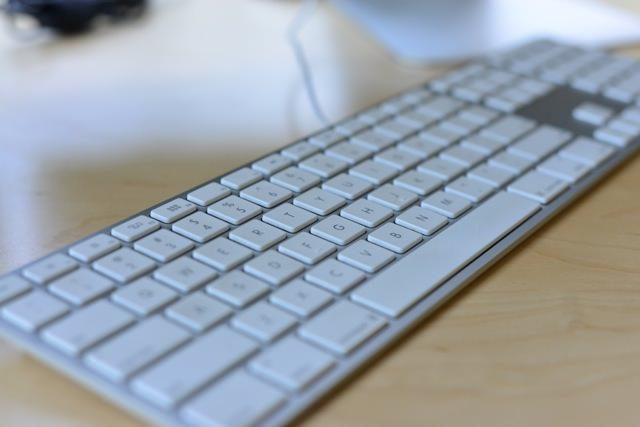
Endertech Senior Project Manager Brian Miller authored this article with minor editing by Digital Media Coordinator Casey. Learn more about our website development services. Contact us for a free consultation.
Top Photo Source: Yuko Honda under license