Add a background image with TailwindCSS
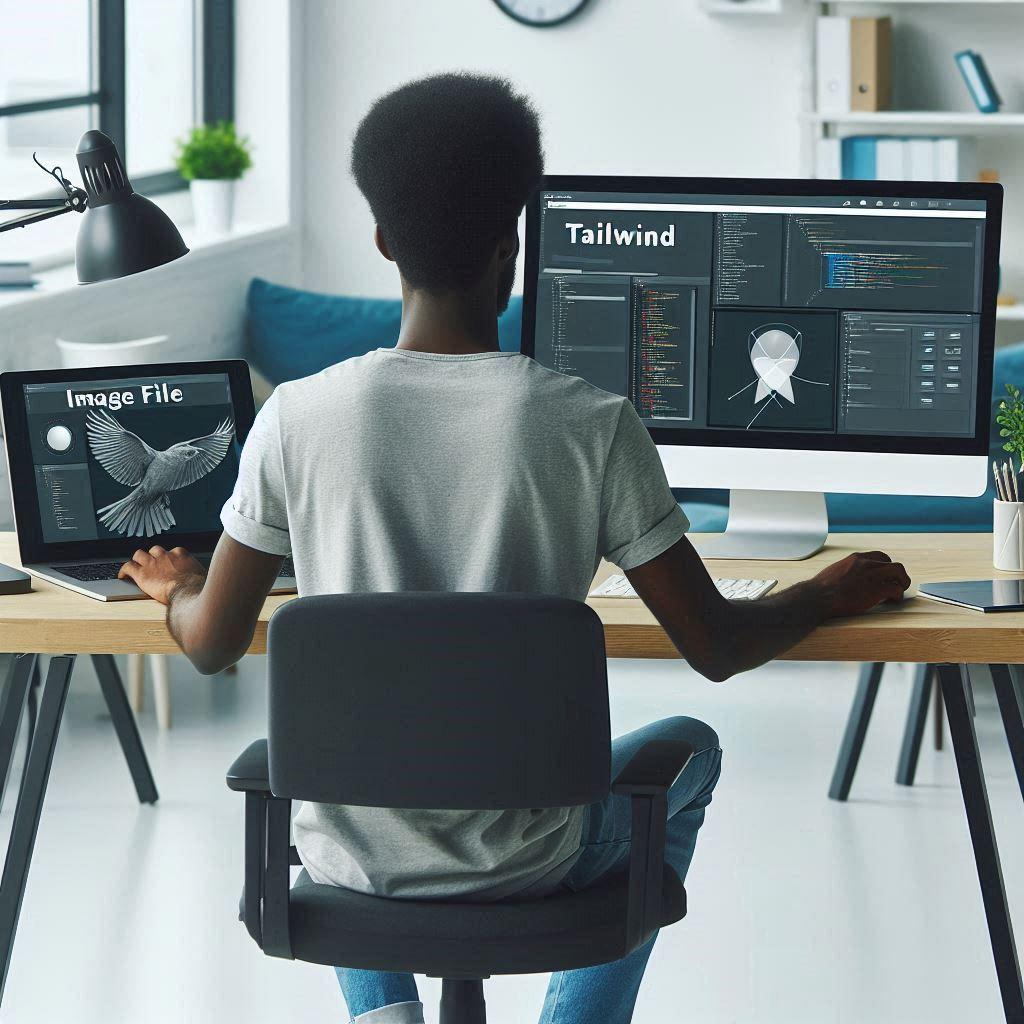
How Tailwind generates CSS
TailwindCSS is a utility-first CSS framework. It focuses on minimizing the final CSS file size by providing prebuilt CSS classes which covers all CSS rules.
Tailwind scans files in a project and generates a CSS file only containing used rules.
When Tailwind scans the below line, it detects three Tailwind classes: "text-3xl", "font-bold", and "underline".
<div class="
text-3xl font-bold underline">
Arbitrary values
Tailwind also allows us to use arbitrary values to create custom classes.
For example, this square bracket notation
<div class="bg-[#001122]">
will generate this class definition:
.bg-\[\#001122\]
background: #001122;
}
Image URL in style definition
Using an image for a <div> background poses a unique challenge when the URL of the image is not available for Tailwind to scan at the build time.
To have a background image for a <div>, we want to have a CSS rule like this:
.my-background-001 {
background-image: url(https://placehold.co/300x500);
}
With the bracket notation, we could try this format:
<div className="bg-[image:url(https://placehold.co/300x500)]">
Replacing the image URL as a variable will result in this code:
<div className={`bg-[image:url(${ imageURL })]`}>
However, Tailwind cannot scan the above line of code because the variable "imageURL" is not available at the build time.
Using CSS variables to embed dynamic values
To workaround the issue, we can use the CSS variables.
We define a CSS variable at an inline style property and use it at the class property placed inside the bracket notation:
<div
style="--image-url: url(https://placehold.co/300x500);"
class="bg-[image:var(--image-url)]"
>
Tailwind is able to generates the below CSS definition:
.bg-\[image\:var\(--image-url\)\]
background-image: var(—image-url);
}
By using CSS variables and the square bracket notation, we can pass variables to Tailwind.
url() does not work with CSS variables
In the above code example, the image URL is placed inside the url() CSS function:
--image-url: url(https://placehold.co/300x500);
We have to include the CSS function url() in the CSS variable definition. Trying to define the variable without the url() will result in the code below, and it does not work:
<div
style="--image-url: https://placehold.co/300x500"
class="bg-[image:url(var(--image-url))]"
>
var() gets escaped
url() is a CSS function which expects a web resource address as its argument.
The argument of the url() function is var(--image-url), and its parenthesis have to be escaped to be a valid web address. The escaped version becomes this:
url( var\(--image-url\) )
However, the escaped parenthesis makes the var() function syntax invalid.
We have to include url() in the CSS variable definition to work with the var() function.